07 Mar 2010 | net |
edit
In the last project I have been working i finally got a chance to design and implement a solution based on NServiceBus and NHibernate, two tools I’ve been watching for a while but never got a chance to play with in more than sample applications. For some external reasons I've been forced to use MySQL as a database server in this project.
So basically I’m using NServiceBus to provide reliable communication between the involved components and NHibernate to do the persistence of the domain objects used by the components. Up to this point the whole design of the solution looks good, with minimal effort i have reliable, fault tolerant services that are ready to do their job.
Now i start implementing the details and get to the point where MySQL comes into play. I must say, it has surprised me... both ways.
The good thing is that after careful tuning, where my previous UNIX experience had a very important role, the database is able to handle the amounts of data that i plan to throw at it. Also it surprised me that some pretty complex queries run a lot faster than expected.
Then the bad things started to show up. The hardest to debug was that updating an indexed column from multiple parallel transactions causes deadlocks witch cause transactions to be aborted. Of course this only happens at high loads. It was not hard to avoid this after i found out what the problem was ... but still after this i had a feeling of working with something that might not be as reliable as expected.
After that the MySQL .NET connector dropped the bomb on me: Distributed Transactions are not supported. Ok, they are not supported but why the hell does the connector throws an exception when used in a distributed transaction? I can understand that i can’t rely on the MySQL transaction being enlisted in the Distributed Transaction (DT) and that i have to handle that myself but not being able to use the connector AT ALL under a DT was unexpected. At this point i see only one solution: grab the source for the connector and modify the part that checks if a DT is present and just ignore it. Turns out this was very easy to do. If anyone is interested in this change in the connector i can provide more details.
Now i need to find a way of having a NHibernate ISession and a ITransaction per NServiceBus message handler.
The first approach was something similar to what Andreas Öhlund describes in this blog post. The only problem is that IMessageModule implementations in NServiceBus 2.0 are singletons and that was a problem because i need to store the ITransaction to commit or rollback at the end of the message handler. If in the next version of the NServiceBus there will be a way to have some message handler “wrapper” that it could work.
My solution was to use a base abstract class for the massage handlers. So instead of just implementing IMessageHandler now i derive from this base class. The code below should speak for itself:
1 /// <summary>
2 /// Base class for message handlers.
3 /// Manages the unit of work required for handling the message.
4 /// </summary>
5 public abstract class MessageHandler<T> : IMessageHandler<T>
6 where T : IMessage
7 {
8 /// <summary>
9 /// The injected unit of work implementation.
10 /// </summary>
11 private readonly IUnitOfWork unitOfWork;
12
13 public MessageHandler(IUnitOfWork unitOfWork)
14 {
15 this.unitOfWork = unitOfWork;
16 }
17
18 /// <summary>
19 /// Concrete handlers must implement this method.
20 /// </summary>
21 public abstract void HandleMessage(T message);
22
23 public void Handle(T message)
24 {
25 try
26 {
27 HandleMessage(message);
28 unitOfWork.Complete();
29 }
30 catch
31 {
32 unitOfWork.Abort();
33 throw;
34 }
35 finally
36 {
37 unitOfWork.Dispose();
38 }
39 }
40 }
The implementation for the IUnitOfWork is in this case very simple, providing only the creation of the session and the transaction and the required operations. Since the unit of work is created per handler and the handlers don’t use other threads to do the work I don’t need to worry about making it thread safe.
1 public class MessageUnitOfWork: IUnitOfWork
2 {
3 private readonly ISessionFactory factory;
4 private readonly ITransaction transaction;
5 private readonly ISession session;
6
7 public MessageUnitOfWork(ISessionFactory factory)
8 {
9 this.factory = factory;
10 session = factory.OpenSession();
11 CurrentSessionContext.Bind(session);
12 transaction = session.BeginTransaction();
13 }
14
15 public void Complete()
16 {
17 transaction.Commit();
18 }
19
20 public void Abort()
21 {
22 transaction.Rollback();
23 }
24
25 public void Dispose()
26 {
27 transaction.Dispose();
28 CurrentSessionContext.Unbind(factory);
29 session.Dispose();
30 GC.SuppressFinalize(this);
31 }
32 }
There is still one small problem. If the handler finishes it’s work without and exception and the mysql transaction is committed BUT an exception is thrown by the bus when committing the distributed transaction the MySQL transaction is not rolled back. But i realized that this only means that the same message might be sent again to the handler and that the handlers in general should handle this logical case since whoever sent the message is free to send it multiple times.
Since I’ve got this solution working it has handled a few millions of messages and there have been crashes and transaction that got rolled back occasionally but after all the system is designed to be fault tolerant and it has proven it is. Also in all the cases the database remained in a consistent state, witch in the beginning i was not sure it will.
In the end i would like to thank the NServiceBus team ( mainly Udi Dahan and Andreas Öhlund ) witch was very responsive and helpful on the support mailing list. I can only hope to find the time to contribute a few ideas to the next release of NSB. Also i would like to thank the NHibernate team for the great product they created ( can’t wait for the 3.0 ).
21 Nov 2009 | net |
edit
A few years ago I've written an article on how to perform a synchronous HTTP request using Qt 4.2. I I've seen this article today and since now I'm mostly working with C# i was wandering how fast can i do the same thing in c#. After very few minutes I've managed to came up with this:
1 using System;
2 using System.Net;
3 using System.IO;
4
5 class Program
6 {
7 static void Main(string[] args)
8 {
9 WebRequest request = HttpWebRequest.Create("http://www.google.com");
10 WebResponse response = request.GetResponse();
11 using(StreamReader reader = new StreamReader(response.GetResponseStream()))
12 Console.WriteLine(reader.ReadToEnd());
13 Console.ReadKey();
14 }
15 }
And of course the WebReqest class allows you to specify all the properties you would ever need for any request, and also allows you to perform request in an asynchronous maner. Now i don't think you can be more expressive than this. I like qt a lot... but i love c# now.
Update...
Or you can do this:
1 using System;
2 using System.Net;
3 using System.IO;
4
5 class Program
6 {
7 static void Main(string[] args)
8 {
9 var content = new WebClient().DownloadString("http://www.google.com");
10 Console.WriteLine(content);
11 }
12 }
11 Jul 2009 | net |
edit
The last 6-7 months i've been working mostly with C# and to be honest I've grown found to it. It has really nice features like lambda expressions, auto properties, extension methods, LINQ witch can really speed up things in terms development time.
Still ... there are some things from C++ that i now miss: Templates ( as in true generics ) , const correctness, deterministic destruction, static initialization and most of all the control over what is a value and what is a reference.
Generics ( Templates )
Yes, C# has generics witch give you a level of freedom and ability to write generic code but they are nothing compared to the power of c++ templates. In C++ templates are compiled when instantiated and you don't need to specify any constraints on the generic type and as long as the instantiated type is syntactically correct your code will compile. There are ways to define concepts witch can impose requirements on the types but the concepts are also verified when the template is instantiated. Also C# does not allow specialization or partial specialization of generic types.
Const Correctness
There are a lot of articles on the web about why C# does not implement const correctness. In the end you learn to leave without it but its a nice thing to have and as long as you use it consistently it can help prevent a lot of bugs mostly design bugs. I guess that there are ways in C# to prevent the bugs that const correctness prevents ( immutable objects, ReadOnly interfaces ) but they are harder to impose on a team of developers.
Deterministic Destruction
I'm quite sure that I only miss this feature because i come with a C++ background. You can easily have deterministic destruction in C# by using IDisposable and using(...) features, still after years of C++ i kinda like to know when an object gets destructed.
Static Initialization
To be honest I don't miss this feature too much. The only thing i find it useful for is implementing the factory pattern where objects register themselves in the factory using static initialization. The downside of this is that it can relay get messy ( hint Static Initialization Fiasco )
Control over what is a value and what is a reference
Now this i miss the most. I have to admit it I like pointers. Well i actually like smart pointers and i like being in control of what and how i pass as arguments to methods. In C# it's written in stone: structs are values and classes are references (ok, with boxing you can have references to structs but that's not the point ). One of the problems is that there is no way of declaring a method that accepts a CONST reference to an instance. When you pass an instance of a class to a method (you actually pass the reference to that instance) , the method has full control over the instance. This might not be a problem when you write all the code, but in a team with developers of different skill bad things can happen. Add some multi-threading to this and things can get relay messy. Another problem is when you have a value object implemented as a struct that is holding a lot of data and you need to pass this object to a Method that only needs to read something from this object. If you pass it as a value object it will probably get copied ( i'm not sure if the compiler does not implement some copy-on-write here but i would not relay on that ) and if you pass it as a ref than the method might change the data.
In conclusion I like working with C# but there are some things that i hope will get better in time. Other important "bonuses" you get with C# are the .NET Framework and Visual Studio IDE that speed up substantially the development. The major downsite witch in some cases is a showstopper: Platform Dependent. I don't know where MONO is right now but if you develop with C# and .NET you better make sure your main target platform is Windows.
I would be glad to hear what others think about this.
26 Jun 2009 | jokes |
edit
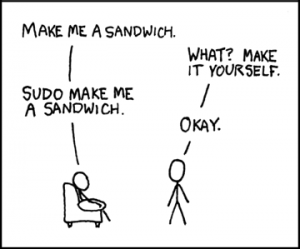
24 Jun 2009 | net |
edit
After a few months I've enjoyed the newly ( or not ) added extension methods in 3.0 yesterday i discovered that one of the assumptions I've made about them was false. My assumption was that if the instance on witch you call an extension method is NULL you would get a NullReferenceException. Turns out it's not the case and you can call the extension method on a null reference.
Thinking about it later, it makes sense. In reality extension methods are nothing more than syntax sugar for calling static methods. And since too much sugar can hurt extension methods might do the same thing if not properly used. The code speaks pretty much for itself.
The class used as example:
1 public class TestClass
2 {
3 //existing method defined on the class
4 public string Hello()
5 {
6 return "Hello";
7 }
8 }
Static class defining extension method
1 public static class ExtensionContainer
2 {
3 //the extension method defined as static
4 public static string NewHello(this TestClass instance)
5 {
6 return "World";
7 }
8 }
Test code:
1 class Program
2 {
3 static void Main(string[] args)
4 {
5 // declare and create a test instance
6 TestClass first = new TestClass();
7
8 Console.WriteLine(first.Hello()); //outputs: "Hello"
9 //call the extension method as a normal method
10 Console.WriteLine(first.NewHello()); // outputs: "World"
11
12 // declare a null reference
13 TestClass second = null;
14
15 try
16 {
17 //calling a method on a null reference throws
18 Console.WriteLine(second.Hello()); //will throw Null Reference exception
19 }
20 catch (NullReferenceException)
21 {
22 Console.WriteLine("NullReference calling Hello");
23 }
24
25 try
26 {
27 //calling an extension method does not throw
28 Console.WriteLine(second.NewHello()); //will not throw
29 }
30 catch (NullReferenceException)
31 {
32 Console.WriteLine("NullReference calling NewHello");
33 }
34
35 //the next two calls are exactly the same
36 Console.WriteLine(second.NewHello());
37 Console.WriteLine(ExtensionContainer.NewHello(second));
38
39 Console.ReadKey();
40 }
41 }
Even now is still consider extension methods as a very valuable addition to the language, but you just need to understand exactly what they are. The wrong assumption about calling them on a null reference can affect some design choices you may take where in case of a null reference you want an exception to be thrown and no other method called.
Also using them when appropriate the ability to call extension methods on null references might help you write more readable code by moving the null check in the extension method. This can be particularly useful on writing extension methods that are supposed to be used in Views in ASP.NET MVC where moving the null check in the method leaves the the view's html code easier to read an understand.
One conclusion that I've drown from this is that in an extension method you should either check for null and throw NullReferenceException if null is not a valid value or handle null appropriately.
PS: Is it visible that the last 6+ months I've been working with C# and not C++ ? :)